The glTexParameter()
function is a crucial part of OpenGL texture mapping, this function determines
the behavior and appearance of textures when they are rendered. Below
is a summary of the various parameters and what their effect is on the
final rendered texture. Take note that each texture uploaded can have
its own separate properties, texture properties are not global. One texture
could clamp is texture coordinates while another wraps them, the properties
of one texture will not effect others.
Target
|
Specifies
the target texture
|
GL_TEXTURE_1D
|
One dimensional
texturing.
|
GL_TEXTURE_2D
|
Two dimensional
texturing.
|
Texture Parameter
|
Accepted values
|
Description
|
GL_TEXTURE_MIN_FILTER
|
GL_NEAREST
, GL_LINEAR, GL_NEAREST_MIPMAP_NEAREST, GL_LINEAR_MIPMAP_NEAREST, GL_NEAREST_MIPMAP_LINEAR
and GL_LINEAR_MIPMAP_LINEAR
|
The texture
minification function is used when a single screen pixel maps to more
than one texel, this means the texture must be shrunk in size.
Default setting
is GL_NEAREST_MIPMAP_LINEAR.
|
GL_TEXTURE_MAG_FILTER
|
GL_NEAREST
or GL_LINEAR
|
The texture
magnification function is used when the pixel being textured maps to an
area less than or equal to one texel, this means the texture must be magnified.
Default setting
is GL_LINEAR.
|
GL_TEXTURE_WRAP_S
|
GL_CLAMP or
GL_REPEAT
|
Sets the wrap
parameter for the s texture coordinate. Can be set to either GL_CLAMP
or GL_REPEAT.
Default setting
is GL_REPEAT.
|
GL_TEXTURE_WRAP_T
|
GL_CLAMP or
GL_REPEAT
|
Sets the wrap
parameter for the t texture coordinate. Can be set to either GL_CLAMP
or GL_REPEAT.
Default setting
is GL_REPEAT.
|
GL_TEXTURE_BORDER_COLOR
|
Any four values
in the [0, 1] range
|
Sets the border
color for the texture, if border is present.
Default setting
is (0, 0, 0, 0).
|
GL_TEXTURE_PRIORITY
|
[0, 1]
|
Specifies
the residence priority of the texture, use to prevent OpenGL from swapping
textures out of video memory. Can be set to values in the [0, 1] range.
See glPrioritizeTextures()
for more information or this article
on Gamasutra.
|
Parameter
|
Description
|
GL_CLAMP
|
Clamps the
texture coordinate in the [0,1] range.
|
GL_REPEAT
|
Ignores the
integer portion of the texture coordinate, only the fractional part is
used, which creates a repeating pattern. A texture coordinate of 3.0 would
cause the texture to tile 3 times when rendered.
|
GL_NEAREST
|
Returns the
value of the texture element that is nearest (in Manhattan distance) to
the center of the pixel being textured. Use this parameter if you would
like your texture to appear sharp when rendered.
|
GL_LINEAR
|
Returns the
weighted average of the four texture elements that are closest to the
center of the pixel being textured. These can include border texture elements,
depending on the values of GL_TEXTURE_WRAP_S and GL_TEXTURE_WRAP_T, and
on the exact mapping. Use this parameter if you would like your texture
to appear blurred when rendered.
|
GL_NEAREST_MIPMAP_NEAREST
|
Chooses the
mipmap that most closely matches the size of the pixel being textured
and uses the GL_NEAREST criterion (the texture element nearest to the
center of the pixel) to produce a texture value.
|
GL_LINEAR_MIPMAP_NEAREST
|
Chooses the
mipmap that most closely matches the size of the pixel being textured
and uses the GL_LINEAR criterion (a weighted average of the four texture
elements that are closest to the center of the pixel) to produce a texture
value.
|
GL_NEAREST_MIPMAP_LINEAR
|
Chooses the
two mipmaps that most closely match the size of the pixel being textured
and uses the GL_NEAREST criterion (the texture element nearest to the
center of the pixel) to produce a texture value from each mipmap. The
final texture value is a weighted average of those two values.
|
GL_LINEAR_MIPMAP_LINEAR
|
Chooses the
two mipmaps that most closely match the size of the pixel being textured
and uses the GL_LINEAR criterion (a weighted average of the four texture
elements that are closest to the center of the pixel) to produce a texture
value from each mipmap. The final texture value is a weighted average
of those two values.
|
Examples
|
Min / Max
Filter : GL_NEAREST
|
Min / Max
Filter : GL_LINEAR
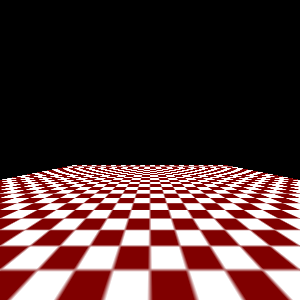
|
Wrap S : GL_CLAMP
Wrap T : GL_CLAMP
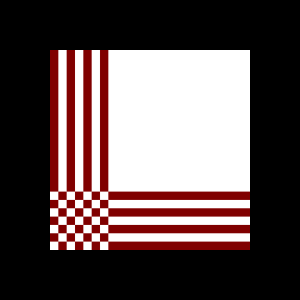
|
Wrap S : GL_CLAMP
Wrap T : GL_REPEAT
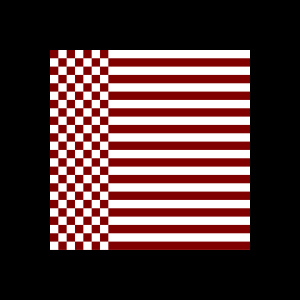
|
Wrap S : GL_REPEAT
Wrap T : GL_CLAMP
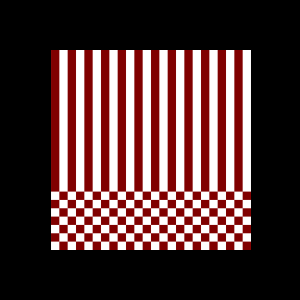
|
Wrap S : GL_REPEAT
Wrap T : GL_REPEAT
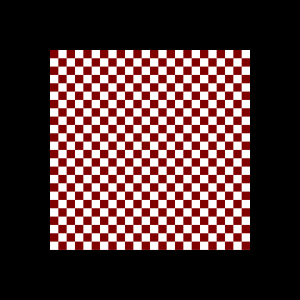
|
|